Python3 Bottleフレームワーク入門(その3)- ORM Peewee (MySQL)
2017年8月24日Python Bottle Framework, トピックス
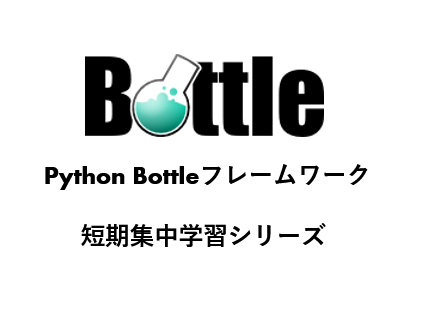
今回はデータベースを使ったケースを学んでいきましょう。フレームワークでの入門編ではほとんどのケースサンプルで載っているのは、Sqlite3を使ったケースが多いですが、実際の運用を考えて実験するとやはりMySQLでの実験運用が現実的と考える。やはり微妙に書き方が変わる可能性があるためそうした方が良いと思います。
また、今の時代フレームワークでORMを使うのが当たり前になってきているためBottleで利用できるORMの一つpeeweeを紹介したいと思います。やはりORMの利用で飛躍的にDBのCRUD処理が楽ちんになります。
peeweeの導入
peeweeを導入するにあたり今回はMySQLを使用した前提で説明していきたいと思います。
必要なMYSQLドライバーを事前にpipやbrewなどで導入してください。必要ドライバー
- MySQL-python
- PyMySQL
1 |
$ pip3 install peewee |
peeweeを導入できたら最初にコードを作る前にMySQLでテーブルを作って見ましょう。既に既存で存在するテーブルからコードを生成する機能が用意されています。
1 2 3 4 5 6 7 8 9 10 11 12 |
CREATE DATABASE IF NOT EXISTS `user_db`; USE `user_db`; CREATE TABLE IF NOT EXISTS `users` ( `id` int(11) NOT NULL AUTO_INCREMENT, `name` varchar(30) NOT NULL, `passwd` varchar(50) NOT NULL, `role` tinyint(4) NOT NULL, PRIMARY KEY (`id`), UNIQUE KEY `name` (`name`) ) ; |
上記のようなデータベースをMySQLで作成してみましょう。このコードをexport.sqlとして保存し、次のようにしてテーブル作成すると簡単で良いと思います。
1 |
$ mysql -u root -p < export.sql |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
$ mysql -u root -p Enter password: Welcome to the MariaDB monitor. Commands end with ; or \g. Your MariaDB connection id is 4 Server version: 10.1.25-MariaDB MariaDB Server Copyright (c) 2000, 2017, Oracle, MariaDB Corporation Ab and others. Type 'help;' or '\h' for help. Type '\c' to clear the current input statement. MariaDB [(none)]> use user_db Reading table information for completion of table and column names You can turn off this feature to get a quicker startup with -A Database changed MariaDB [user_db]> show tables; +-------------------+ | Tables_in_user_db | +-------------------+ | user | +-------------------+ 1 row in set (0.00 sec) MariaDB [cole_db]> desc user; +--------+-------------+------+-----+---------+----------------+ | Field | Type | Null | Key | Default | Extra | +--------+-------------+------+-----+---------+----------------+ | id | int(11) | NO | PRI | NULL | auto_increment | | name | varchar(30) | NO | UNI | NULL | | | passwd | varchar(50) | NO | | NULL | | | role | tinyint(4) | NO | | NULL | | +--------+-------------+------+-----+---------+----------------+ 4 rows in set (0.00 sec) |
1 |
$ python -m pwiz -e mysql -H localhost -u root -P user_db > models.py |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
from peewee import * database = MySQLDatabase('user_db', **{'user': 'root', 'host': 'localhost', 'password': 'test01'}) class UnknownField(object): def __init__(self, *_, **__): pass class BaseModel(Model): class Meta: database = database class User(BaseModel): name = CharField(unique=True) passwd = CharField() role = IntegerField() class Meta: db_table = 'user' |
DBから特定ユーザデータを抜き出して表示するプログラムを見てみましょう。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
from bottle import mako_view as view from bottle import Bottle,route,run,template from peewee import * database = MySQLDatabase('user_db', **{'user': 'root', 'host': 'localhost', 'password': 'test01'}) class UnknownField(object): def __init__(self, *_, **__): pass class BaseModel(Model): class Meta: database = database class User(BaseModel): name = CharField(unique=True) passwd = CharField() role = IntegerField() class Meta: db_table = 'user' app=Bottle() database.connect() @app.route('/user/:name') @view('userinfo') def userall(name): query = User.get(User.name == name) return {'name':query.name,'password':query.passwd,'role':query.role} @app.route('/hello/:names') @view('hello') def index(names): return {'name':names} if __name__ == "__main__": run(app=app,host="0.0.0.0",port='8080',debug=True) |
1 2 3 4 5 6 |
<%inherit file="layout/base.html"/> <%block name="header"> <strong>名前は${name}</strong></p> <strong>パスワードは${password}</strong></p> <strong>ロールは${role}</strong></p> </%block> |
実際にブラウザからhttp://xxx.xxx.xxx.xxx:8080/user/James というようにチェックするためには、テーブルにダミーデータを入れておく必要があります。
◯ テストデータをDBへインサート
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
from peewee import * database = MySQLDatabase('user_db', **{'user': 'root', 'host': 'localhost', 'password': 'test01'}) class UnknownField(object): def __init__(self, *_, **__): pass class BaseModel(Model): class Meta: database = database class Users(BaseModel): name = CharField(unique=True) passwd = CharField() role = IntegerField() class Meta: db_table = 'users' database.connect() #テストデータをテーブルへインサート data1=Users(name='kazuo',passwd='test0123',role=2) data2=Users(name='hanako',passwd='test78484',role=1) data3=Users(name='masako',passwd='ddgdfhdhd',role=0) data1.save() data2.save() data3.save() database.close() |
これで全ての準備が整ったので、改めてブラウザからhttp://xxx.xxx.xxx.xxx:8080/user/kazuoでアクセスしてみましょう。下記のように表示されたら成功です。
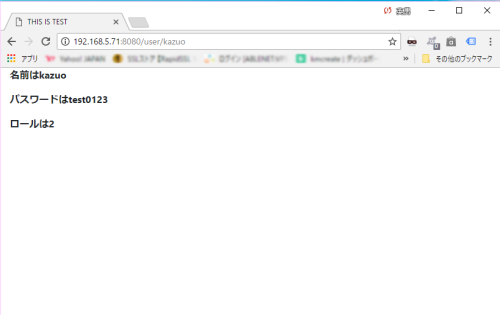
- Python Bottle Framework入門 全13 回
- 1.基礎編サーバ起動
- 2.リクエストメソッド
- 3.ORM Peewee (MySQL)
- 4.ORM Peewee CRUD
- 5.Cookie And Session
- 6.Abort and Redirect
- 7.マルチスレッドWEBサーバ
- 8.デーモン化
- 9.Json
- 10.WSGI on SSL
- 11.Apache連携起動(外部WSGI) SSL接続
- 12.Apache連携起動(ReverseProxy)SSL接続
- 13.hprox連携起動(ReverseProxy)SSL接続&HTTP2対応