マルチFormページでの個別Form毎のボタンをjavascriptでイベントスキャンし対応する。
2021/05/30
2021/11/24
タグ: javascript, jQuery, マルチForm, 個別対応, 無効化
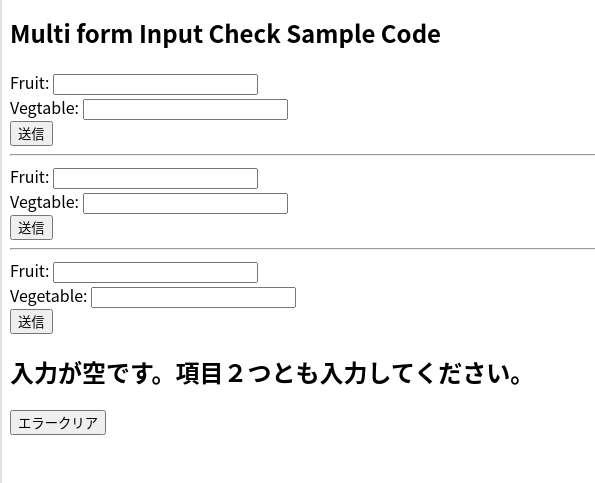
講習でアプリ開発を行わせている傍ら、受講生から1ページに複数のFormエントリーが存在していた場合どうやって個別にjavascriptで制御したらよいか質問された。 普段あまりjavascriptをコーディングする機会がないため、即答できず考え方の説明でその場は終わったが 改めて時間を作って実験してみた。 今回はjQueryを使わない方法と使う方両方でやってみました。 テストなのでactionのスクリプトは指定なしとしました。
サンプルHTMLページ
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="utf-8"> <title>Javascript Sample</title> </head> <!-- <body onload="printMoji()"> --> <body> <h2>Multi form Input Check Sample Code </h2> <form id="form1" action="" method="post" > <label>Fruit: <input type="text" class="inp01" name="fruit" ></label><br> <label>Vegtable: <input type="text" class="inp02" name="vegtable" ></label><br> <input type="submit" onClick="btn_check('form1');"> </form> <hr> <form id="form2" action="" method="post" > <label>Fruit: <input type="text" class="inp01" name="fruit" ></label><br> <label>Vegetable: <input type="text" class="inp02" name="vegtable" ></label><br> <input type="submit" onClick="btn_check('form2');"> </form> <hr> <form id="form3" action="" method="post" > <label>Fruit: <input type="text" class="inp01" name="fruit" ></label><br> <label>Vegetable: <input type="text" class="inp02" name="vegtable" ></label><br> <input type="submit" onclick="btn_check('form3');"> </form> <h2 id="output"></h2> <button>エラークリア</button> <script src="test04.js"></script> </body> </html> |
javascriptのコード
e.preventDefault();を入れないと直ぐにエラーメッセージの表示が消えてなくなるため使用します。この機能はaction=””でやると自分自身のページに 戻るためリロードをかけた状態になりエラーメッセージが直ぐに消えてなくなるための対処です。つまりエラーが生じたときはsubmitさせないための処置。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
function btn_check(form_id){ const id_filter = "#" + form_id; let form = document.querySelector(id_filter); form.addEventListener('submit', function(e) { let text1 = document.querySelector(id_filter + " .inp01"); let text2 = document.querySelector(id_filter + " .inp02"); if( text1.value == "" || text2.value == ""){ e.preventDefault(); result = "入力が空です。項目2つとも入力してください。"; document.getElementById('output').innerHTML = result; return false; } return true; }); } let button1 = document.querySelector('button'); button1.addEventListener('click', function() { document.getElementById('output').innerHTML = ""; }); |
jQueryを使った場合のHTMLコード
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
<!DOCTYPE html> <html lang="ja"> <head> <meta charset="utf-8"> <title>Coffee Script</title> <style> .cols { color:#A93226; font-size:26px; } </style> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> </head> <body> <h2>Sample script test (jQuery)</h2> <form id="form1" action="" method="post"> <label>Fruit: <input type="text" class="inp01" name="fruit" ></label><br> <label>Vegtable: <input type="text" class="inp02" name="vegtable" ></label><br> <input type="submit" onClick="btnCheck('form1')"> </form> <hr> <form id="form2" action="" method="post"> <label>Fruit: <input type="text" class="inp01" name="fruit" ></label><br> <label>Vegtable: <input type="text" class="inp02" name="vegtable" ></label><br> <input type="submit" onClick="btnCheck('form2')"> </form> <hr> <form id="form3" action="" method="post"> <label>Fruit: <input type="text" class="inp01" name="fruit" ></label><br> <label>Vegtable: <input type="text" class="inp02" name="vegtable" ></label><br> <input type="submit" onClick="btnCheck('form3')"> </form> <script src="test03.js"></script> </body> </html> |
jQueryを使った場合のjavascriptコード
1 2 3 4 5 6 7 8 9 10 11 12 13 |
function btnCheck(id){ form = "#" + id; $(form).submit(function(e) { text_input1 = document.querySelector(form + " .inp01"); text_input2 = document.querySelector(form + " .inp02"); if( text_input1.value == "" || text_input2.value == ""){ e.preventDefault(); alert("入力がありません。"); return false; } return true; }); } |